Offering discounts on orders with a status like “Processing” or “On Hold” is a strategic move to clear payment pending orders, especially during sales or promotional periods. For example, when customers abandons an order without making a payment, offering a 10% discount will encourage customers to continue their purchase, knowing they’re getting a better deal.
Solution: Implement Order Status-based discounts
The code snippet will apply a 10% discount to on-hold order status, and it will display the discount information accordingly on the order received page and the customer’s account page.
function ts_apply_discount_on_order_on_hold( $order_id ) { // Get the order object $order = wc_get_order( $order_id ); // Check if the order status is "On Hold" if ( $order && $order->get_status() === 'on-hold' ) { // Calculate discount amount (e.g., $10 off for orders on hold) $discount_amount = 10; // Example: $10 discount // Apply discount to order total $order->set_total( $order->get_total() - $discount_amount ); // Save the order $order->save(); // Add discount details to order meta for reference $order->update_meta_data( 'discount_amount', $discount_amount ); $order->save_meta_data(); } } add_action( 'woocommerce_order_status_on-hold', 'ts_apply_discount_on_order_on_hold', 10, 1 ); /** * Display discount information on the order received page. */ function ts_display_discount_information_on_thank_you_page( $order_id ) { // Get the order object $order = wc_get_order( $order_id ); // Get discount amount from order meta $discount_amount = $order->get_meta( 'discount_amount' ); // Display discount information on the order received page if ( $discount_amount ) { echo '<p><strong>Discount Applied:</strong> $' . $discount_amount . '</p>'; } } add_action( 'woocommerce_thankyou', 'ts_display_discount_information_on_thank_you_page', 10, 1 ); /** * Display discount information on the customer's account page. */ function ts_display_discount_information_on_account_page( $order ) { // Get discount amount from order meta $discount_amount = $order->get_meta( 'discount_amount' ); // Display discount information on the customer's account page if ( $discount_amount ) { echo '<p><strong>Discount Applied:</strong> $' . $discount_amount . '</p>'; } } add_action( 'woocommerce_order_details_after_order_table', 'ts_display_discount_information_on_account_page', 10, 1 );
Output
When customers view their order details and see the discount applied, they’re more likely to proceed with the purchase.
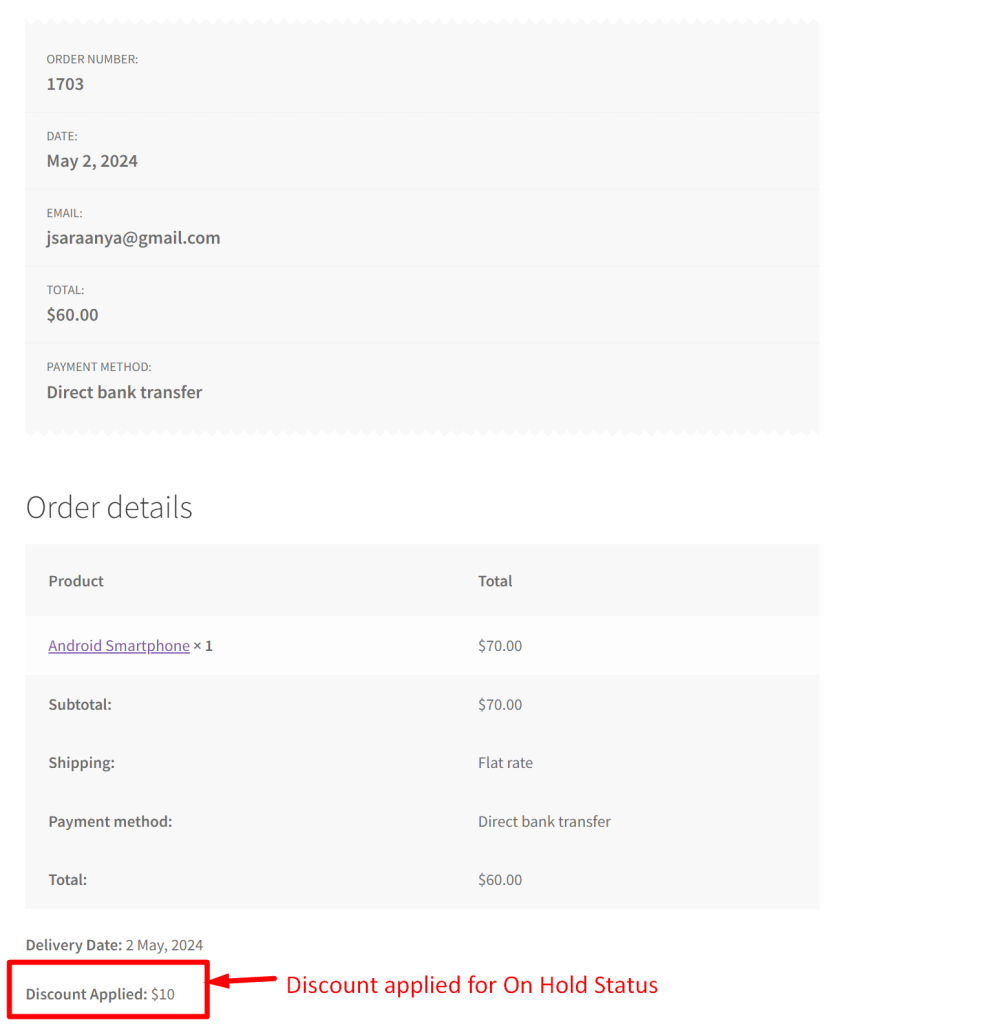
The same discount that is applied for orders with specific status on hold is also shown on the My Account page.
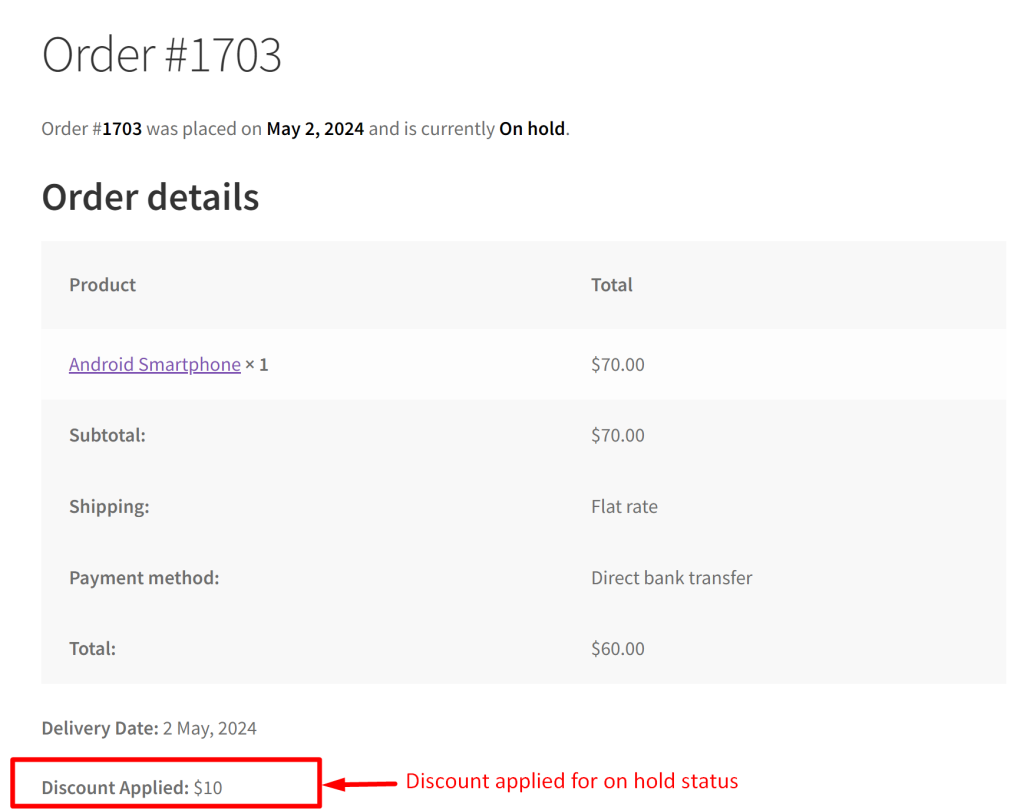
Want to enhance your order status based promotion by providing a free gift instead of a discount? Check out this post that will help you add a bogo buy one get one offer on WooCommerce custom order status change.